Angular is a popular open-source JavaScript framework used for building dynamic and feature-rich web applications. It was developed by Google and was first released in 2010. Angular is a component-based framework that follows the Model-View-Controller (MVC) architectural pattern, which separates the application logic from the user interface.
**Key Features of Angular:**
* Two-way data binding: Angular automatically updates the view whenever there is a change in the model, and vice versa.
* Dependency injection: Angular provides a built-in dependency injection system that simplifies the process of managing dependencies between components.
* Reactive programming: Angular supports reactive programming using RxJS, which allows developers to build complex and asynchronous applications.
* Routing and navigation: Angular provides a powerful routing mechanism that enables developers to create single-page applications with multiple views and routes.
* Form handling: Angular offers a robust form handling mechanism that simplifies the process of creating and managing forms in web applications.
**Use Cases of Angular:**
Angular is widely used for building complex and feature-rich web applications, such as:
Advertisement
* E-commerce websites
* Social media platforms
* Online marketplaces
* Enterprise applications
* Content management systems
**2. Understanding Angular Components**
In Angular, a component is the fundamental building block of an application. It is a self-contained unit that encapsulates a specific functionality and can be reused across the application. Components are composed of three parts:
* Template: It defines the structure and layout of the component using HTML.
* Class: It defines the behavior and logic of the component using TypeScript.
* Metadata: It provides additional information about the component, such as its selector, inputs, and outputs.
**Creating an Angular Component:**
To create an Angular component, follow these steps:
1. Generate a new component using the Angular CLI command ng generate component component-name.
2. Define the component\'s template using HTML.
3. Define the component\'s class using TypeScript.
4. Add the component to a module using the @NgModule decorator.
**3. Angular Directives**
Directives in Angular are used to add behavior or modify the appearance of elements in the DOM. There are three types of directives:
* Structural directives: They modify the structure of the DOM, such as ngIf, ngFor, and ngSwitch.
* Attribute directives: They modify the appearance or behavior of an element, such as ngClass, ngStyle, and ngModel.
* Component directives: They are used to create reusable components.
**Creating a Custom Directive:**
To create a custom directive in Angular, follow these steps:
1. Generate a new directive using the Angular CLI command ng generate directive directive-name.
2. Define the directive\'s logic using TypeScript.
3. Add the directive to a module using the @NgModule decorator.
4. Apply the directive to an element in the template using its selector.
**4. Angular Services**
Services in Angular are used to provide a way to share data or functionality across different components in an application. They are singletons, meaning that there is only one instance of a service per application.
**Creating an Angular Service:**
To create an Angular service, follow these steps:
1. Generate a new service using the Angular CLI command ng generate service service-name.
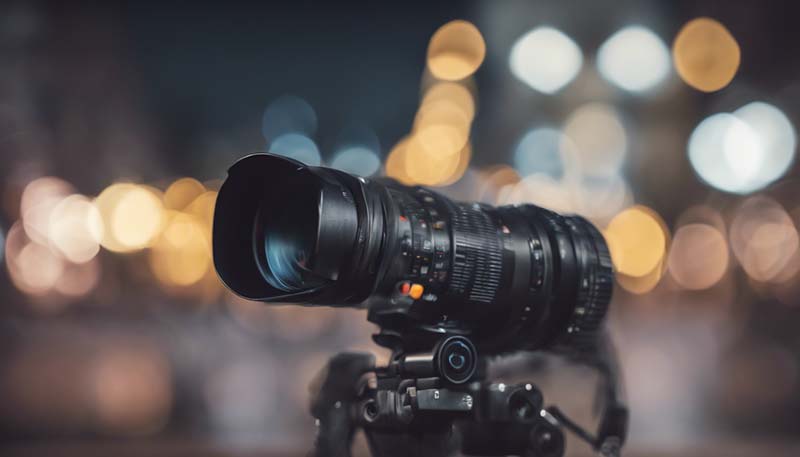
2. Define the service\'s logic using TypeScript.
3. Add the service to a module using the @NgModule decorator.
4. Inject the service into a component using the constructor and dependency injection.
**5. Angular Modules**
Modules in Angular are used to organize and encapsulate related components, directives, and services. They define a namespace for the application and can be lazy-loaded to improve performance.
**Creating an Angular Module:**
To create an Angular module, follow these steps:
1. Generate a new module using the Angular CLI command ng generate module module-name.
2. Define the module\'s logic using TypeScript.
3. Add components, directives, and services to the module using the @NgModule decorator.
4. Import the module into the application using the AppModule\'s imports array.
**6. Angular Forms**
Forms in Angular are used to collect and validate user input. There are two types of forms:
* Template-driven forms: They use HTML form elements and Angular directives to create and validate forms.
* Reactive forms: They use a programmatic approach to create and validate forms using the FormBuilder service and FormGroup and FormControl classes.
**Creating an Angular Form:**
To create an Angular form, follow these steps:
1. Choose between template-driven or reactive forms based on your requirements.
2. Define the form\'s structure and logic using HTML for template-driven forms or TypeScript for reactive forms.
3. Add form controls and validation rules to the form.
4. Bind the form to a component using two-way data binding or a reactive approach.
**7. Angular Routing**
Routing in Angular is used to navigate between different views in a single-page application. Angular provides a powerful routing mechanism that enables developers to define routes and navigate between them.
**Configuring Angular Routing:**
To configure Angular routing, follow these steps:
1. Import the RouterModule and Routes class from @angular/router.
2. Define the application\'s routes using the Routes class.
3. Add the RouterModule to the AppModule\'s imports array.
4. Use the RouterLink directive to navigate between routes.
5. Use the RouterOutlet directive to display the selected route\'s component.
**8. Angular Animations**
Animations in Angular are used to add visual effects to components and make the user interface more engaging. Angular provides a comprehensive animation library that enables developers to create complex animations using a simple and intuitive API.
**Creating Angular Animations:**
To create Angular animations, follow these steps:
1. Import the BrowserAnimationsModule from @angular/platform-browser/animations.
2. Add the BrowserAnimationsModule to the AppModule\'s imports array.
3. Define the animation\'s keyframes and timing using the @keyframes rule in CSS.
4. Bind the animation to a component using the [@trigger] syntax.
5. Control the animation\'s state using the ngAnimate directives, such as ngIf, ngFor, and ngSwitch.
**9. Angular Material**
Angular Material is a UI component library that provides a set of pre-built, customizable, and responsive components based on Google\'s Material Design guidelines. It is designed to work seamlessly with Angular and can be easily integrated into any Angular application.
**Using Angular Material Components:**
To use Angular Material components, follow these steps:
1. Install Angular Material using the Angular CLI command ng add @angular/material.
2. Import the desired Angular Material modules, such as MatButtonModule or MatInputModule, into the application\'s module.
3. Add the Angular Material components to the application\'s template using their respective selectors.
4. Customize the components\' appearance using CSS or Sass.
**10. Conclusion**
Angular is a powerful and versatile framework that enables developers to build complex and feature-rich web applications. By understanding the key concepts and components of Angular, such as directives, services, modules, forms, routing, animations, and Angular Material, you can create engaging and interactive web applications that provide a great user experience.
Leave a Reply